Trim Component
#
Getting StartedThe below code will give you the full Trim Component within your application to play with.
note
type="disabled"
only disabled processing, that is when you press the done button.
The rest of the component is fully fucntionally
You can find an interactive demo at api.modfy.video/components
#
ProcessingYou can learn the basics of processing here
Specific to the Trim
component, the callback function in other(change to manual)
mode is:
You can use this to manually trim the video if you want to
#
ThemesExamples of themes are shown below
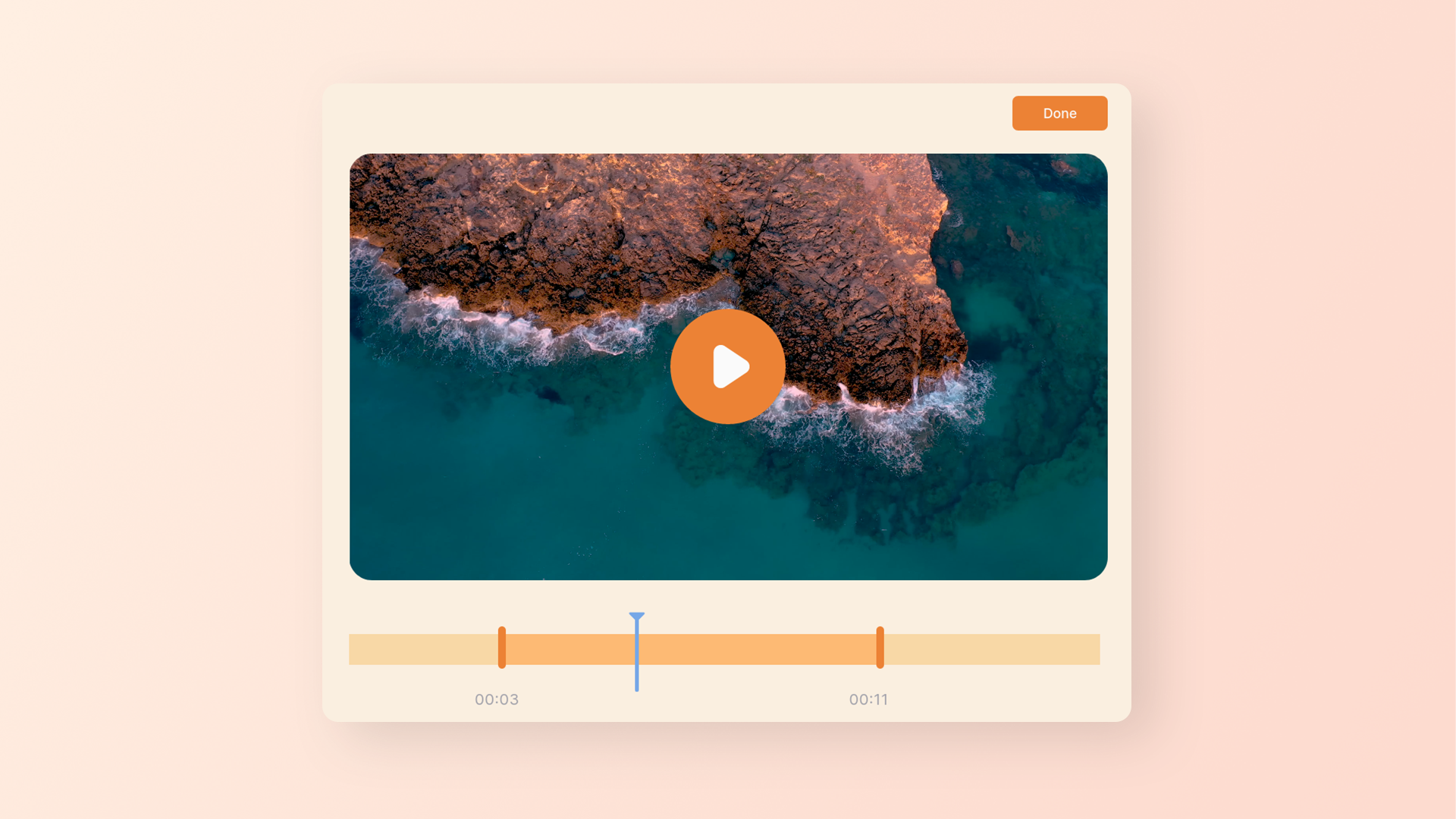
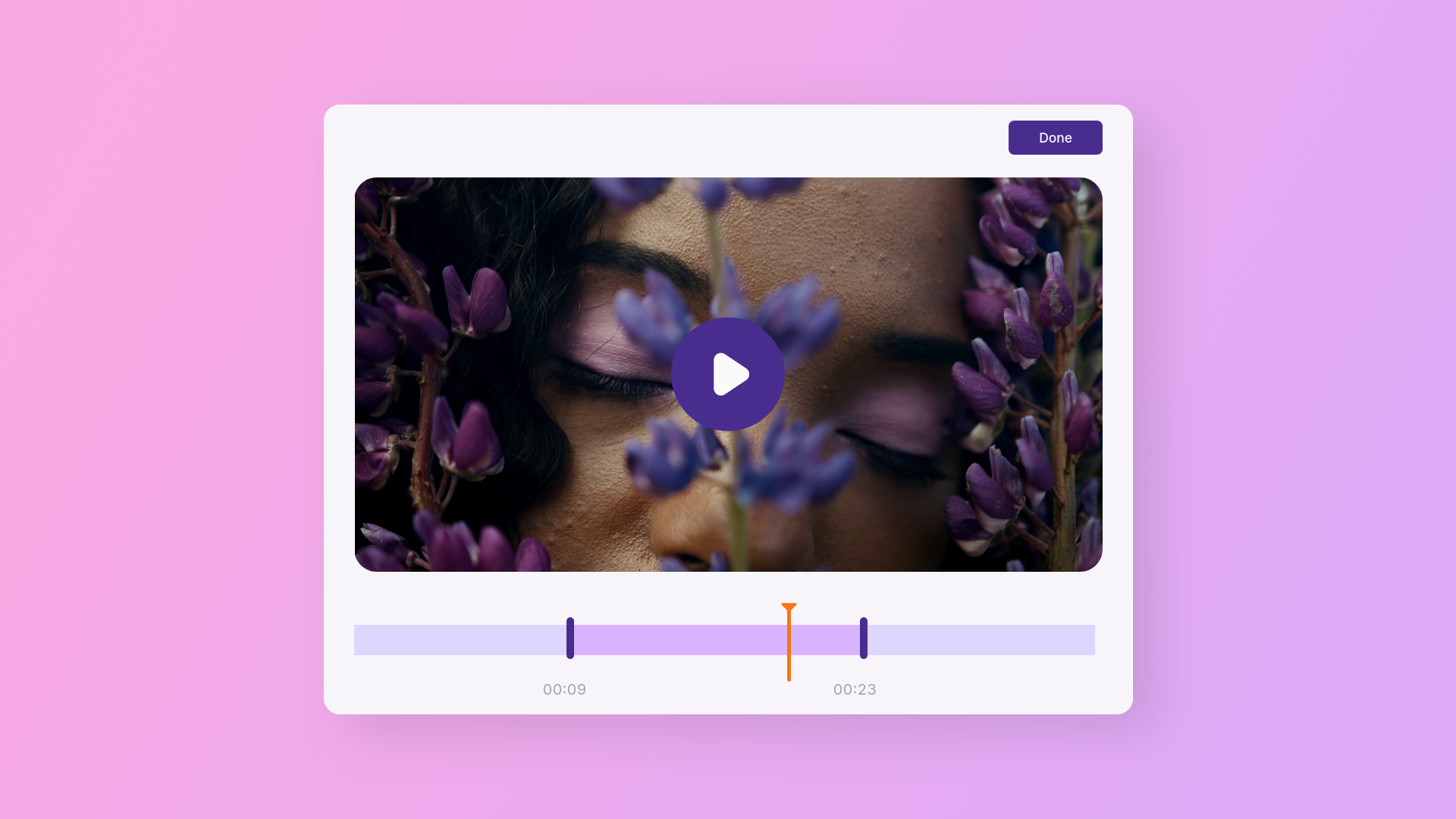
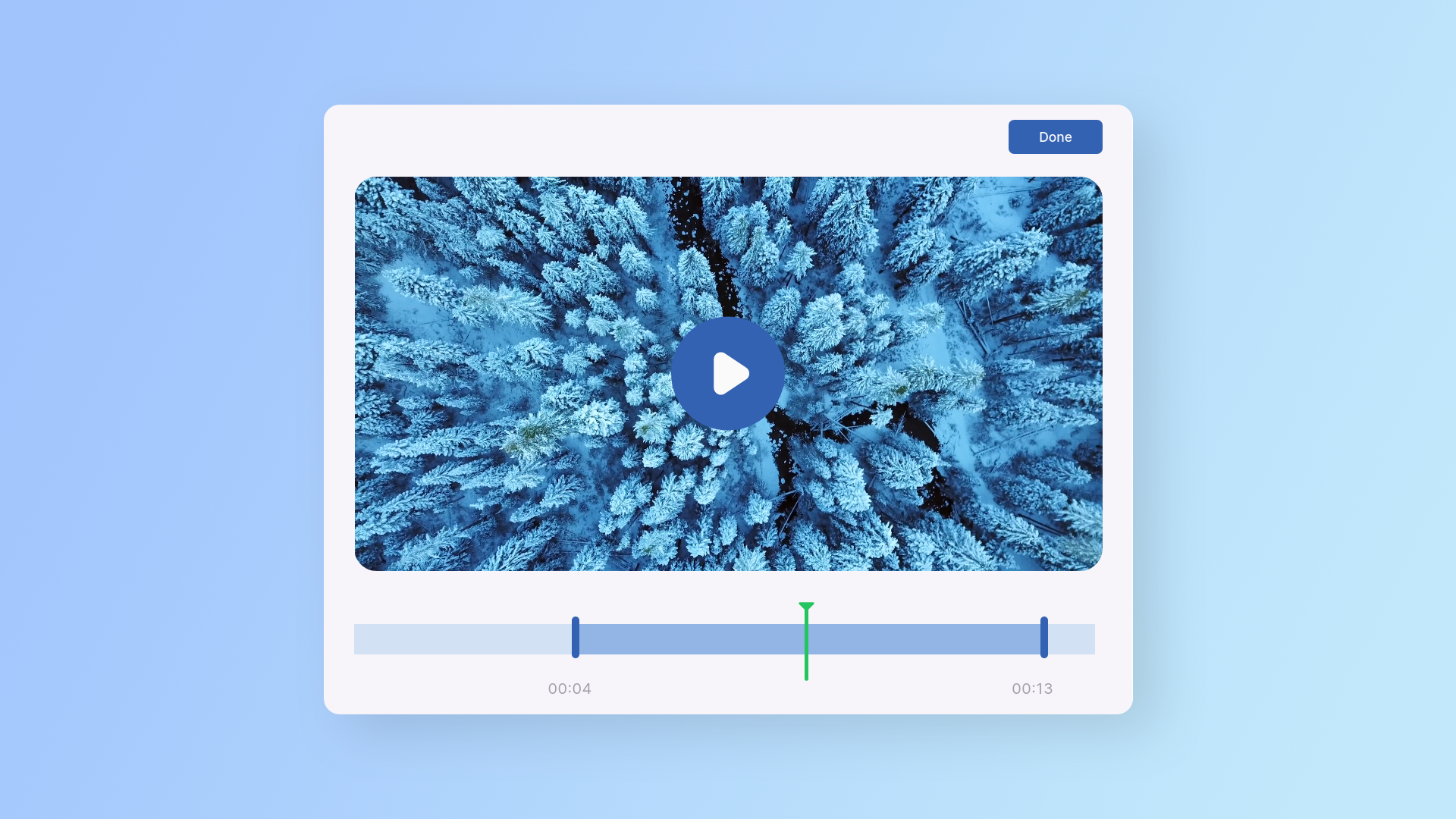
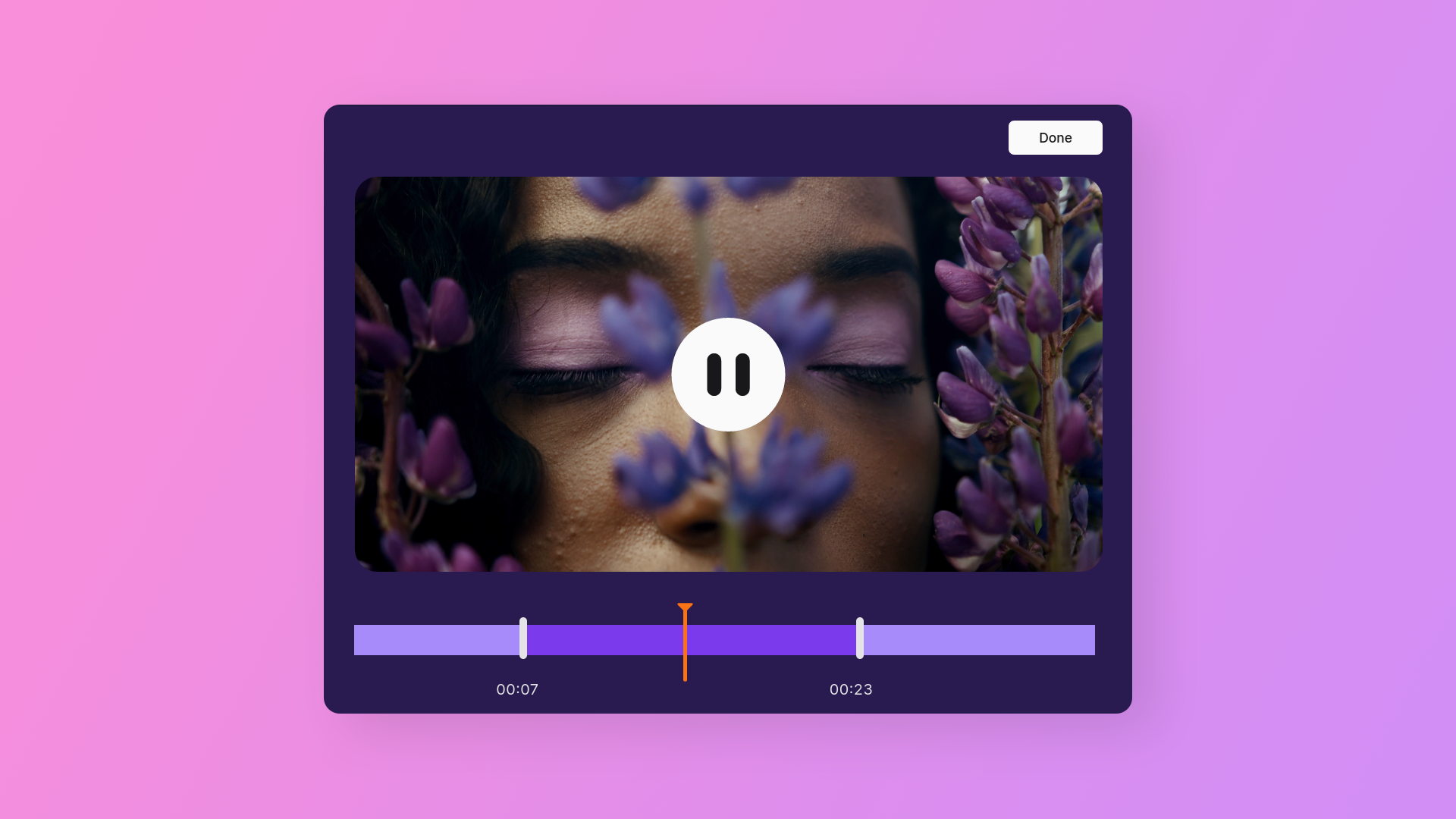
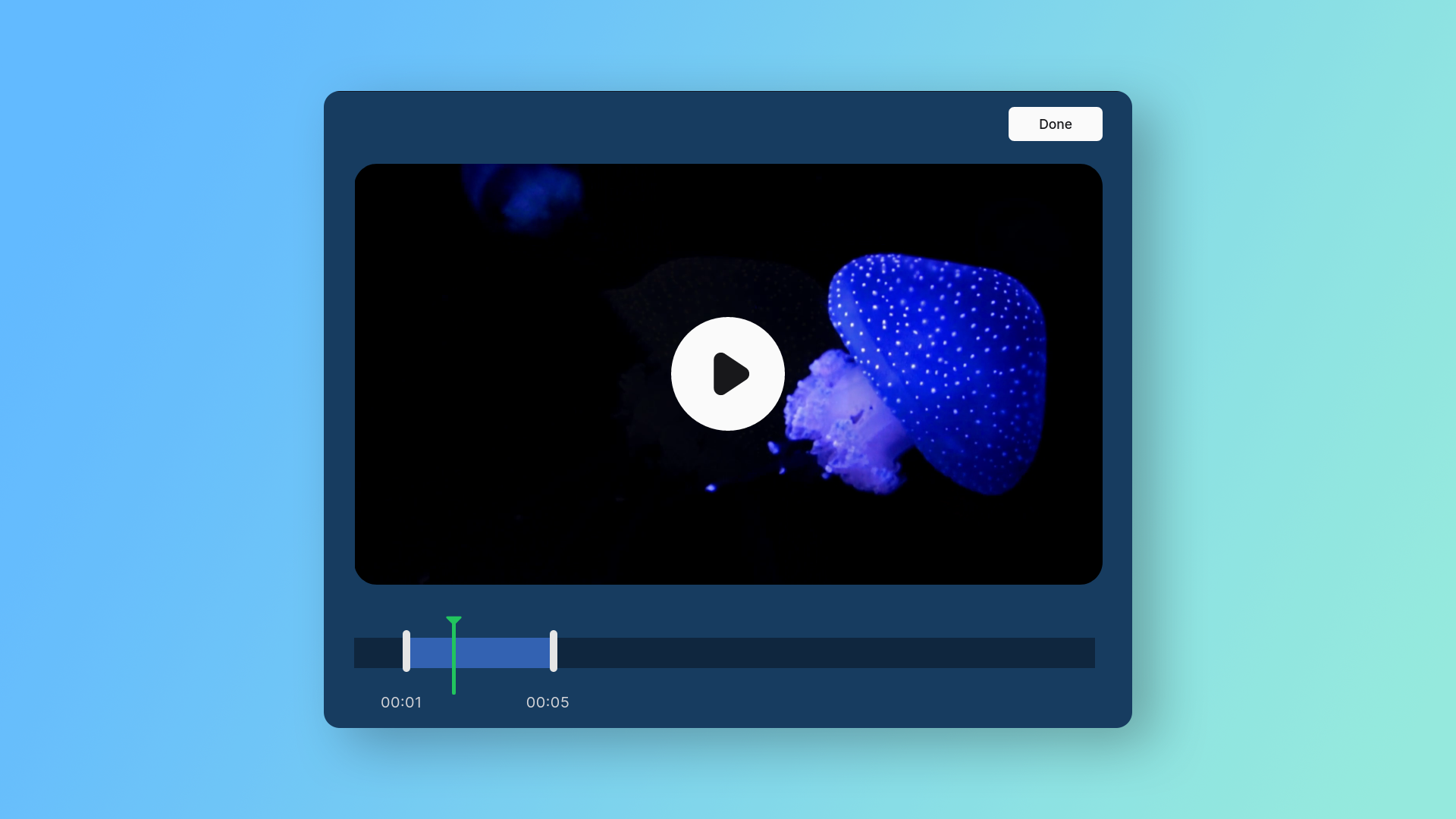
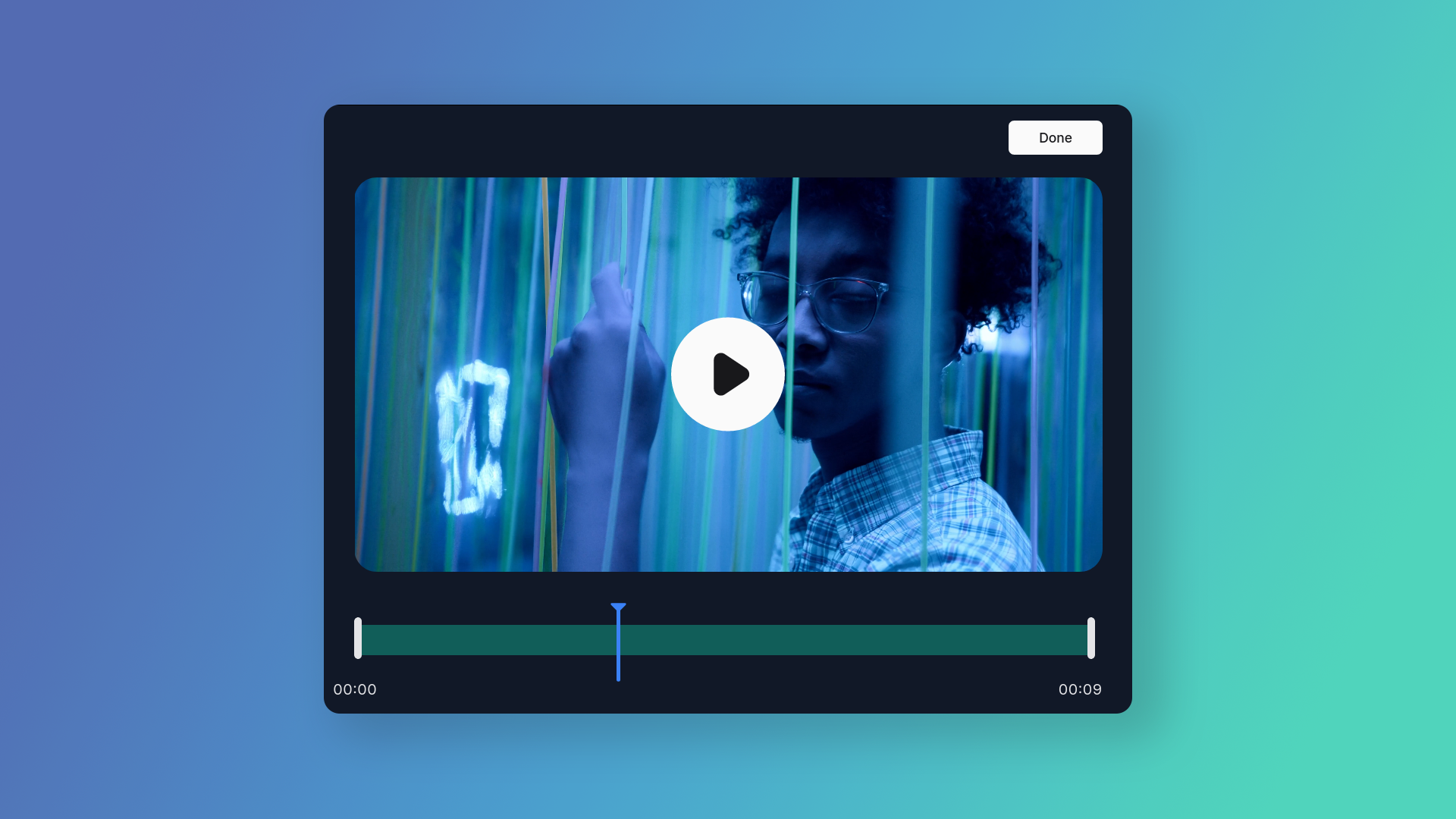
#
Light Themes- Autumn
- Amethyst
- Lapis
#
Dark Themes- Atlantis
- Amethyst Dark
- Lapis Dark
#
Custom ThemesYou can easily customize the color of all the elements using custom themes
- Extend a theme you like or defaultTheme
- Update the colors you want to change
- Use theme
These are the following properties you can customize in your themes
#
CustomizationThe below are the full range of customizable properties for style.
text
The text object just contains the text you can edit or modifyfont
By default, the font used isInter
you can overwrite it and useinherit
An example of some basic customization would be